본문
180905(수) - Google Maps (Map Objects)
Google Maps
Map Objects
Note : Maps SDK requires API level 12 or higher for support of MapFragment
만약 application 이 API level 12 이하이면 SupportMapFragment를 대신 사용
Note : getMapAsync() 는 반드시main thread에서 called & callback도 마찬가지로 main thread에서 executed
Google Play services가 깔려있지 않는 user's device는 Play services가 installs 되기 전까지 callback이 triggered되지 않는다.
@Override
public void onMapReady(GoogleMap map) {
map.addMarker(new MarkerOptions()
.position(new LatLng(0, 0))
.title("Marker"));
}
The map object
- Google Maps for Mobile(GMM)이라는게 있으며, Google Maps for Android와는 비슷하긴 하지만 다르다.
・ API가 displayed하는 Map tiles에는 personalized smart icons같은 content가 없다.
・ Map의 all icons는 non-clickable
・ 대신 map에 add된 marker는 clickable, 다양한 listener callback API 제공
・ 이외에 Android UI model과 interactions 가능한 full range API support
- GoogleMap은 application 내부에서 map model를 object화 한다.
- UI에서 GoogleMap은 MapFragment or MapView object로 represented된다.
- GoogleMap은 다음을 automatically 처리 한다.
・ Connecting to the Google map service
・ Downloading map tiles
・ Displaying tiles on device screen
・ Displaying various controls (ex. pan and zoom)
・ Responding to pan and zoom gestures by moving the map and zooming in or out
- 이외의 동작은 objects and method of API를 통해서 control 가능하다.
MapFragment
- subclass of Fragment
- map에 대한 containers 역할
- GoogleMap object에 access할 수 있도록 provide
- Activity에서 user interface의 behavior or potion을 represents
- Single activity에서 multiple fragment를 combine 하여 build multi-pane UI
- Reuse fragment in multiple activity
MapView
- MapView
- Subclass of View
- MapFragment와 비슷하게 map에 대한 container역할을 하며, GoogleMap object의 core functionality를 exposing
- API를 fully interactive mode로 사용한다면, MapView class must forward following activity lifecycle
ex. onCreate(), onStart(), onResume(), onPause(), onStop(), onDestroy(), onSaveInstanceStats(), onLowMemory()
- API를 lite mode로 사용한다면, forwarding lifecycle은 optional
Map types
- map types of maps 존재
- map's type이 overall representation을 제어
ex) atlas usually contains political maps (focus on showing boundaries, show all roads or city or region)
Normal
- road map
- road, some built by humans, important natural features like rivers
- road, feature labels visible
Hybrid
- satellite photograph data with road map
- road, feature labels visible
Satellite
- satellite photograph data
- road, feature labels not visible
Terrain
- Topographic data
- colors, contour lines, labels, perspective shading
- some roads and labels are visible
None
- no tiles
- tile이 loaded 되지 않은 empty grid 로 rendering
Change the map type
GoogleMap map;
...
// Sets the map type to be "hybrid"
map.setMapType(GoogleMap.MAP_TYPE_HYBRID);
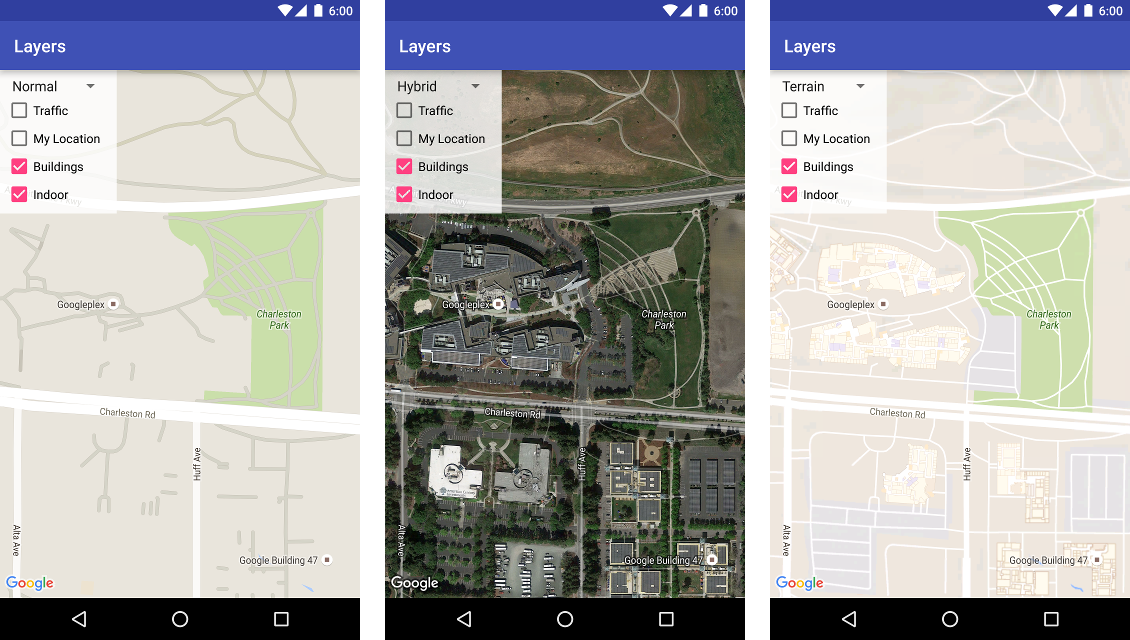
Indoor maps
- high zoom levels에서 map shows floor plans
ex) airport, shopping malls, large retail stores, transit stations
- Indoor map이라고 불리는 이 floor plans는 Normal and Satellite map type에서 displayed 된다.
- user zooms in, and fade away 일 때, automatically enabled 된다.
Deprecation notice : future release, indoor maps는 only normal map type에서만 사용 가능하다.
Work with indoor maps in the API
- default, indoor map enabled
- indoor map은 on map at a time이다.
- second map에 indoor enable을 하고 싶다면, first map을 disable 먼저 해야한다.
- default level picker를 disable하고 싶으면, GoogleMap.getUiSettings().setIndoorLevelPickerEnabled(false)
- IndoorBuilding
and IndoorLevel
- Styling of the base map은 indoor map에 영향이 없다.
Add floor plans
- directly Add floor plans
- display ground overlay or tile overlay
Configure initial state
- application's needs에 맞게 initial state configure를 설정 가능
・ camera position, including
- location, zoom, bearing and tilt
・ map type
・ zoom buttons / compass appear or not
・ manipulate the camera on user gesture
・ lite mode enabled or not
Using XML attributes
- Maps API 는 MapFragment or MapView initialize를 위한 custom XML attributes를 제공
- zOrderOrTop
・ map view's surface가 window top에 위치할지 아닌지 결정
・ SurfaceView.setZOrderOnTop(boolean)
- useViewLifeCycle
・ only valid MapFragment
・ default value == false, tying the lifecycle of map to fragment
・ here
xmlns:map="http://schemas.android.com/apk/res-auto"
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:map="http://schemas.android.com/apk/res-auto"
android:name="com.google.android.gms.maps.SupportMapFragment"
android:id="@+id/map"
android:layout_width="match_parent"
android:layout_height="match_parent"
map:cameraBearing="112.5"
map:cameraTargetLat="-33.796923"
map:cameraTargetLng="150.922433"
map:cameraTilt="30"
map:cameraZoom="13"
map:mapType="normal"
map:uiCompass="false"
map:uiRotateGestures="true"
map:uiScrollGestures="false"
map:uiTiltGestures="true"
map:uiZoomControls="false"
map:uiZoomGestures="true"/>
Note : Google Maps APIs Premium Plan license를 사용중이라면, attribute name prefix에 m4b_ 를 붙여라.
Programmatically
- 옵션은 XML과 동일
GoogleMapOptions options = new GoogleMapOptions();
options.mapType(GoogleMap.MAP_TYPE_SATELLITE)
.compassEnabled(false)
.rotateGesturesEnabled(false)
.tiltGesturesEnabled(false);
- MapFragment.newInstance(GoogleMapOptions options)
- MapView(Context, GoogleMapOptions)
Map padding
- camera's target은 padding region의 center
- legal information, copyright statement, google logo 등이 edge에 표시된다.
- map은 전체 container를 채우지만, 안의 action들은 padding region 만큼 줄어든 영역에서 동작한다.
Note : Google Maps Platform Terms of Service에 따라서, application은 google logo or copyright notices를 가리거나 지우면 안된다.
'Mobile > Google Maps' 카테고리의 다른 글
180907(금) - Google Maps (Lite Mode) (0) | 2018.09.07 |
---|---|
180906(목) - Google Maps (Businesses and Other POI) (0) | 2018.09.06 |
180905(수) - Google Maps (Select Current Place) (0) | 2018.09.05 |
180905(수) - Google Maps (Polylines and Polygons to Represent Routes and Areas) (0) | 2018.09.05 |
180904(화) - Google Maps (Map with a Marker) (0) | 2018.09.04 |
댓글